Design the corresponding flowchart and create a trace table to determine the values of the variables in each step of the next program for two different executions.
The input values for the two executions are (i) 10, and (ii) 51.
PHP
<?php
$a = trim(fgets(STDIN));
$y = 5;
if ($a * 2 > 100) {
$a = $a * 3;
$y = $a * 4;
}
echo $a, " ", $y;
?>
Java
public static void main(String[] args) throws java.io.IOException {
java.io.BufferedReader cin = new java.io.
BufferedReader(new java.io.InputStreamReader(System.in));
int a, y;
a = Integer.parseInt(cin.readLine());
y = 5;
if (a * 2 > 100) {
a = a * 3;
y = a * 4;
}
System.out.println(a + " " + y);
}
C++
#include <iostream>
using namespace std;
int main() {
int a, y;
cin >> a;
y = 5;
if (a * 2 > 100) {
a = a * 3;
y = a * 4;
}
cout << a << " " << y;
return 0;
}
C#
static void Main() {
int a, y;
a = Int32.Parse(Console.ReadLine());
y = 5;
if (a * 2 > 100) {
a = a * 3;
y = a * 4;
}
Console.Write(a + " " + y);
Console.ReadKey();
}
Visual Basic
Sub Main()
Dim a, y As Integer
a = Console.ReadLine()
y = 5
If a * 2 > 100 Then
a = a * 3
y = a * 4
End If
Console.Write(a & " " & y)
Console.ReadKey()
End Sub
Python
a = int(input())
y = 5
if a * 2 > 100:
a = a * 3
y = a * 4
print(a, y)
Solution
The flowchart is shown here.
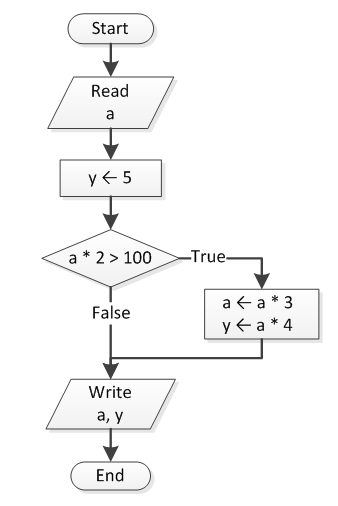
The trace tables for each input are shown here.
i. For the input value of 10, the trace table looks like this.
PHP
Step |
Statement |
Notes |
$a |
$y |
1 |
$a = trim(fgets(STDIN)) |
User enters the value 10 |
10 |
? |
2 |
$y = 5 |
|
10 |
5 |
3 |
if ($a * 2 > 100) |
This evaluates to false |
4 |
echo $a, " ", $y |
The values 10, 5 are displayed |
Java
Step |
Statement |
Notes |
a |
y |
1 |
a = Integer.parseInt(cin.readLine()) |
User enters the value 10 |
10 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
if (a * 2 > 100) |
This evaluates to false |
4 |
System.out.println(a + " " + y) |
The values 10, 5 are displayed |
C++
Step |
Statement |
Notes |
a |
y |
1 |
cin >> a |
User enters the value 10 |
10 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
if (a * 2 > 100) |
This evaluates to false |
4 |
cout << a << " " << y |
The values 10, 5 are displayed |
C#
Step |
Statement |
Notes |
a |
y |
1 |
a = Int32.Parse(Console.ReadLine()) |
User enters the value 10 |
10 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
if (a * 2 > 100) |
This evaluates to false |
4 |
Console.Write(a + " " + y) |
The values 10, 5 are displayed |
Visual Basic
Step |
Statement |
Notes |
a |
y |
1 |
a = Console.ReadLine() |
User enters the value 10 |
10 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
If a * 2 > 100 Then |
This evaluates to false |
4 |
Console.Write(a & " " & y) |
The values 10, 5 are displayed |
Python
Step |
Statement |
Notes |
a |
y |
1 |
a = int(input()) |
User enters the value 10 |
10 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
if a * 2 > 100: |
This evaluates to false |
4 |
print(a, y) |
The values 10, 5 are displayed |
ii. For the input value of 51, the trace table looks like this.
PHP
Step |
Statement |
Notes |
$a |
$y |
1 |
$a = trim(fgets(STDIN)) |
User enters the value 51 |
51 |
? |
2 |
$y = 5 |
|
10 |
5 |
3 |
if ($a * 2 > 100) |
This evaluates to true |
4 |
$a = $a * 3 |
|
153 |
5 |
5 |
$y = $a * 4 |
|
153 |
612 |
6 |
echo $a, " ", $y |
The values 153, 612 are displayed |
Java
Step |
Statement |
Notes |
a |
y |
1 |
a = Integer.parseInt(cin.readLine()) |
User enters the value 51 |
51 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
if (a * 2 > 100) |
This evaluates to true |
4 |
a = a * 3 |
|
153 |
5 |
5 |
y = a * 4 |
|
153 |
612 |
6 |
System.out.println(a + " " + y) |
The values 153, 612 are displayed |
C++
Step |
Statement |
Notes |
a |
y |
1 |
cin >> a |
User enters the value 51 |
51 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
if (a * 2 > 100) |
This evaluates to true |
4 |
a = a * 3 |
|
153 |
5 |
5 |
y = a * 4 |
|
153 |
612 |
6 |
cout << a << " " << y |
The values 153, 612 are displayed |
C#
Step |
Statement |
Notes |
a |
y |
1 |
a = Int32.Parse(Console.ReadLine()) |
User enters the value 51 |
51 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
if (a * 2 > 100) |
This evaluates to true |
4 |
a = a * 3 |
|
153 |
5 |
5 |
y = a * 4 |
|
153 |
612 |
6 |
Console.Write(a + " " + y) |
The values 153, 612 are displayed |
Visual Basic
Step |
Statement |
Notes |
a |
y |
1 |
a = Console.ReadLine() |
User enters the value 51 |
51 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
If a * 2 > 100 Then |
This evaluates to true |
4 |
a = a * 3 |
|
153 |
5 |
5 |
y = a * 4 |
|
153 |
612 |
6 |
Console.Write(a & " " & y) |
The values 153, 612 are displayed |
Python
Step |
Statement |
Notes |
a |
y |
1 |
a = int(input()) |
User enters the value 51 |
51 |
? |
2 |
y = 5 |
|
10 |
5 |
3 |
if a * 2 > 100: |
This evaluates to true |
4 |
a = a * 3 |
|
153 |
5 |
5 |
y = a * 4 |
|
153 |
612 |
6 |
print(a, y) |
The values 153, 612 are displayed |