A car starts from rest and moves with a constant acceleration along a straight horizontal road for a given time. Write a program that prompts the user to enter the acceleration and the time the car traveled, and then calculates and displays the distance traveled. The required formula is
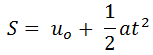
where
- S is the distance the car traveled, in meters (m)
- uo is the initial velocity (speed) of the car, in meters per second (m/sec)
- t is the time the car traveled, in seconds (sec)
- a is the acceleration, in meters per second2 (m/sec2)
Solution
Since the car starts from rest, the initial velocity (speed) u0 is zero. Thus, the formula becomes
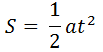
and the program is
PHP
<?php
echo "Enter acceleration: ";
$a = trim(fgets(STDIN));
echo "Enter time traveled: ";
$t = trim(fgets(STDIN));
$S = 0.5 * $a * $t * $t;
echo "Your car traveled ", $S, " meters";
?>
Java
public static void main(String[] args) throws java.io.IOException {
java.io.BufferedReader cin = new java.io.
BufferedReader(new java.io.InputStreamReader(System.in));
double S, a, t;
System.out.print("Enter acceleration: ");
a = Double.parseDouble(cin.readLine());
System.out.print("Enter time traveled: ");
t = Double.parseDouble(cin.readLine());
S = 0.5 * a * t * t;
System.out.println("Your car traveled " + S + " meters");
}
C++
#include <iostream>
using namespace std;
int main() {
double S, a, t;
cout << "Enter acceleration: ";
cin >> a;
cout << "Enter time traveled: ";
cin >> t;
S = 0.5 * a * t * t;
cout << "Your car traveled " << S << " meters";
return 0;
}
C#
static void Main() {
double S, a, t;
Console.Write("Enter acceleration: ");
a = Double.Parse(Console.ReadLine());
Console.Write("Enter time traveled: ");
t = Double.Parse(Console.ReadLine());
S = 0.5 * a * t * t;
Console.Write("Your car traveled " + S + " meters");
Console.ReadKey();
}
Visual Basic
Sub Main()
Dim S, a, t As Double
Console.Write("Enter acceleration: ")
a = Console.ReadLine()
Console.Write("Enter time traveled: ")
t = Console.ReadLine()
S = 0.5 * a * t ^ 2
Console.Write("Your car traveled " & S & " meters")
Console.ReadKey()
End Sub
Python
a = float(input("Enter acceleration: "))
t = float(input("Enter time traveled: "))
S = 0.5 * a * t ** 2
print("Your car traveled", S, "meters")