Every high-level programming language provides many functions that can be used whenever and wherever you wish. Functions are nothing more than a block of statements packaged as a unit that has a name and performs a specific task.
Notice: Not every computer language uses the term “function”. For example Java, C#, Visual Basic, and Python are using the term “method”.
To better understand functions, let’s take Heron’s iterative formula that calculates the square root of a positive number.
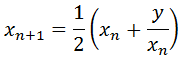
where
- y is the number for which you want to find the square root
- xn is the n-th iteration value of the square root of y
Please don’t be disappointed! No one at the present time calculates the square root of a number this way. Fortunately, high-level programming languages such as PHP, Java, C++, C#, Visual Basic, and Python include a function for that purpose! This function, which is actually a small subprogram, has been given a name, for example sqrt
, and the only thing you have to do is call it by its name and it will do the job for you. This function probably uses Heron’s iterative formula, or perhaps a formula of another ancient or modern mathematician. The truth is that you don’t really care! What really matters is that this function gives you the right result! An example is shown here.
PHP
<?php
$x = trim(fgets(STDIN));
$y = sqrt($x);
echo sqrt($y);
?>
If you need more information you can visit PHP Mathematical Functions
Java
public static void main(String[] args) throws java.io.IOException {
java.io.BufferedReader cin = new java.io.
BufferedReader(new java.io.InputStreamReader(System.in));
double x, y;
x = Double.parseDouble(cin.readLine());
y = Math.sqrt(x);
System.out.println(y);
}
If you need more information you can visit Java Mathematical Methods
C++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double x, y;
cin >> x;
y = sqrt(x);
cout << y;
return 0;
}
Notice: Please note that the function
sqrt()
is defined in the librarycmath
. Therefore, in order to use the functionsqrt
, you must include the librarycmath
using the statement#include <cmath>
at the beginning of your program.
If you need more information you can visit C++ Mathematical Functions
C#
static void Main() {
double x, y;
x = Double.Parse(Console.ReadLine());
y = Math.Sqrt(x);
Console.Write(y);
Console.ReadKey();
}
If you need more information you can visit C# and Visual Basic Mathematical Methods
Visual Basic
Sub Main()
Dim x, y As Double
x = Console.ReadLine()
y = Math.Sqrt(x)
Console.Write(y)
Console.ReadKey()
End Sub
If you need more information you can visit C# and Visual Basic Mathematical Methods
Python
import math
x = float(input())
y = math.sqrt(x)
print(y)
Notice: The method
sqrt()
is not accessible directly in Python, so you need to importmath
module. A module is nothing more than a file that contains many ready-to-use functions (or methods). Python incorporates quite a lot such modules, but, if you wish to use a function or a method included in one of those modules, you need to import that module into your program.
If you need more information you can visit Python Mathematical Methods