A flowchart uses the oblique parallelogram and the reserved word “Write” to display a message or the final results to the user’s screen.
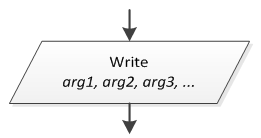
where arg1, arg2, and arg3 can be variables, expressions, or even strings enclosed in double quotes.
The oblique parallelogram that you have just seen is equivalent to
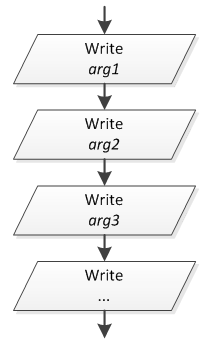
In a computer language, you can achieve the same result by using the statement
PHP
echo arg1, arg2, arg3, … ;
or the equivalent sequence of statements.
echo arg1;
echo arg2;
echo arg3;
…
Java
System.out.print(arg1 + arg2 + arg3 + … );
or the equivalent sequence of statements.
System.out.print(arg1);
System.out.print(arg2);
System.out.print(arg3);
…
C++
cout << arg1 << arg2 << arg3 << … ;
or the equivalent sequence of statements
cout << arg1;
cout << arg2;
cout << arg3;
…
C#
Console.Write(arg1 + arg2 + arg3 + … );
or the equivalent sequence of statements
System.out.print(arg1);
System.out.print(arg2);
System.out.print(arg3);
…
Visual Basic
Console.Write(arg1 & arg2 & arg3 & … )
or the equivalent sequence of statements
Console.Write(arg1)
Console.Write(arg2)
Console.Write(arg3)
…
Python
print(arg1, arg2, arg3, … [, sep = " "] [, end = "\n"])
where
arg1, arg2, arg3, …
are the arguments (values) to be printed. They can be variables, expressions, constant values, or strings enclosed in double quotes.sep
is the string inserted between arguments. It is optional and its default value is one space character.end
is the string appended after the last argument. It is optional and its default value is one “line break.”
Remember! If you want to display a string on the screen, the string must be enclosed in double quotes.
The following program:
PHP
<?php
$a = 5 + 6;
echo "The sum of 5 and 6 is ", $a;
?>
Java
public static void main(String[] args) throws java.io.IOException {
int a;
a = 5 + 6;
System.out.print("The sum of 5 and 6 is " + a);
}
C++
#include <iostream>
using namespace std;
int main() {
int a;
a = 5 + 6;
cout << "The sum of 5 and 6 is " << a;
return 0;
}
C#
static void Main() {
int a;
a = 5 + 6;
Console.Write("The sum of 5 and 6 is " + a);
Console.ReadKey();
}
Visual Basic
Sub Main()
Dim a As Integer
a = 5 + 6
Console.Write("The sum of 5 and 6 is " & a)
Console.ReadKey()
End Sub
Python
a = 5 + 6
print("The sum of 5 and 6 is", a)
display the message shown in Figure 1.
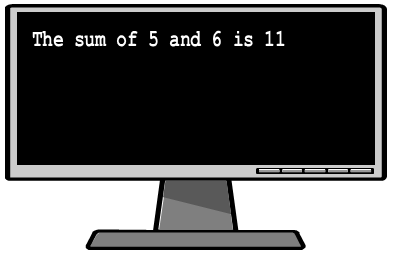
Figure 1 A string displayed on the screen
You can also calculate the result of a mathematical expression directly in an output statement. The following statement:
PHP
<?php
echo "The sum of 5 and 6 is ", 5 + 6;
?>
Java
public static void main(String[] args) throws java.io.IOException {
System.out.print("The sum of 5 and 6 is " + (5 + 6));
}
C++
#include <iostream>
using namespace std;
int main() {
cout << "The sum of 5 and 6 is " << 5 + 6;
return 0;
}
C#
static void Main() {
Console.Write("The sum of 5 and 6 is " + (5 + 6));
Console.ReadKey();
}
Visual Basic
Sub Main()
Console.Write("The sum of 5 and 6 is " & (5 + 6))
Console.ReadKey()
End Sub
Python
print("The sum of 5 and 6 is", 5 + 6)
displays exactly the same message as the statements in Figure 1.