Do you recall the three main stages involved in creating an algorithm or a computer program? The first stage was the “data input” stage, in which the computer lets the user enter data such as numbers, their name, their address, or their year of birth.
A flowchart uses the oblique parallelogram and the reserved word “Read” to let a user enter his or her data.
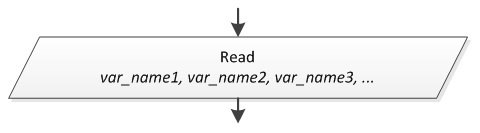
where var_name1, var_name2, and var_name3 must be variables only.
The oblique parallelogram that you have just seen is equivalent to
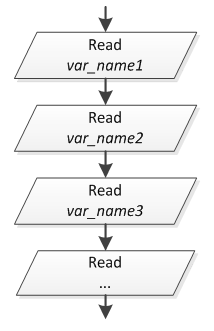
Notice: When a Read statement is executed, the flow of execution is interrupted until the user has entered all the data. When data entry is complete, the flow of execution continues to the next statement. Usually data are entered from a keyboard.
PHP
In PHP, data input is accomplished using the PHP statement
$var_name = trim(fgets(STDIN));
where
$var_name
can be any variable.STDIN
stands for STANDARD INPUT (this is usually the keyboard).- function
trim()
is discussed later.
The following code fragment lets the user enter his or her name and age.
$name = trim(fgets(STDIN));
$age = trim(fgets(STDIN));
echo "Wow, you are already", age, "years old,", name, "!";
It could be even better if, before each data input, a “prompt” message is displayed. This makes the program more user-friendly. For example, look at the following program.
echo "What is your name?";
$name = trim(fgets(STDIN));
echo "What is your age?";
$age = trim(fgets(STDIN));
echo "Wow, you are already", age, "years old,", name, "!";
Java
In Java, data input is accomplished using the following Java statements
java.io.BufferedReader cin = new java.io.
BufferedReader(new java.io.InputStreamReader(System.in));
String var_name_str;
int var_name_int;
long var_name_long;
double var_name_dbl;
//Read a string from the keyboard
var_name_str = cin.readLine();
//Read an integer from the keyboard
var_name_int = Integer.parseInt(cin.readLine());
//Read a long integer from the keyboard
var_name_long = Long.parseLong(cin.readLine());
//Read a real from the keyboard
var_name_dbl = Double.parseDouble(cin.readLine());
where
var_name_str
can be any variable of type String.var_name_int
can be any variable of type int.var_name_long
can be any variable of type long.var_name_dbl
can be any variable of type double.
The following code fragment lets the user enter his or her name and age.
java.io.BufferedReader cin = new java.io.BufferedReader(new java.io.InputStreamReader(System.in));
String name;
int age;
name = cin.readLine();
age = Integer.parseInt(cin.readLine());
System.out.print("Wow, you are already" + age + "years old," + name + "!");
It could be even better if, before each data input, a “prompt” message is displayed. This makes the program more user-friendly. For example, look at the following program.
java.io.BufferedReader cin = new java.io.BufferedReader(new java.io.InputStreamReader(System.in));
String name;
int age;
System.out.print("What is your name? ");
name = cin.readLine();
System.out.print("What is your age? ");
age = Integer.parseInt(cin.readLine());
System.out.print("Wow, you are already" + age + "years old," + name + "!");
C++
In C++, data input is accomplished using the following C++ statements
//Read a number or a string (without spaces or tabs) from the keyboard
cin >> var_name;
//Read a string that includes spaces and tabs from the keyboard
getline(cin, var_name_str);
where
var_name
can be a variable of any type.var_name_str
can be any variable of type string.
Notice: Please note that
cin
considers space characters as terminating characters when reading a string from the keyboard. Thus, reading a string withcin
means to always read a single word, not a phrase or an entire sentence. To read an entire line you can use thegetline()
function.
The statement cin
can also be used to request more than one value. The following code fragment lets the user enter his or her name and age.
string name;
int age;
cin >> name >> age;
cout << "Wow, you are already" << age << "years old," << name << "!");
However, it could be even better if, before each data input, a “prompt” message is displayed. This makes the program more user-friendly. For example, look at the following program.
string name;
int age;
cout << "What is your name? ";
cin >> name;
cout << "What is your age? ";
cin >> age;
cout << "Wow, you are already" << age << "years old," << name << "!");
C#
In C#, data input is accomplished using the following C# statements
string var_name_str;
short var_name_short;
int var_name_int;
long var_name_long;
double var_name_dbl;
//Read a string from the keyboard
var_name_str = Console.ReadLine();
//Read a short integer from the keyboard
var_name_short = Int16.Parse(Console.ReadLine());
//Read an integer from the keyboard
var_name_int = Int32.Parse(Console.ReadLine());
//Read a long integer from the keyboard
var_name_long = Int64.Parse(Console.ReadLine());
//Read a real from the keyboard
var_name_dbl = Double.Parse(Console.ReadLine());
where
var_name_str
can be any variable of type string.var_name_short
can be any variable of type short.var_name_int
can be any variable of type int.var_name_long
can be any variable of type long.var_name_dbl
can be any variable of type double.
The following code fragment lets the user enter his or her name and age.
string name;
byte age;
name = Console.ReadLine();
age = Byte.Parse(Console.ReadLine());
Console.Write("Wow, you are already" + age + "years old," + name + "!");
However, it could be even better if, before each data input, a “prompt” message is displayed. This makes the program more user-friendly. For example, look at the following program.
string name;
byte age;
Console.Write("What is your name? ");
name = Console.ReadLine();
Console.Write("What is your age? ");
age = Byte.Parse(Console.ReadLine());
Console.Write("Wow, you are already" + age + "years old," + name + "!");
Visual Basic
In Visual Basic, data input is accomplished using the following Visual Basic statements
Dim var_name_str As String
Dim var_name_short As Short
Dim var_name_int As Integer
Dim var_name_long As Long
Dim var_name_dbl As Double
'Read a string from the keyboard
var_name_str = Console.ReadLine()
'Read a short integer from the keyboard
var_name_short = Console.ReadLine()
'Read an integer from the keyboard
var_name_int = Console.ReadLine()
'Read a long integer from the keyboard
var_name_long = Console.ReadLine()
'Read a real from the keyboard
var_name_dbl = Console.ReadLine()
where
var_name_str
can be any variable of type String.var_name_short
can be any variable of type Short.var_name_int
can be any variable of type Integer.var_name_long
can be any variable of type Long.var_name_dbl
can be any variable of type Double.
The following code fragment lets the user enter his or her name and age.
Dim name As String
Dim age As Byte
name = Console.ReadLine()
age = Console.ReadLine()
Console.Write("Wow, you are already" & age & "years old," & name & "!")
However, it could be even better if, before each data input, a “prompt” message is displayed. This makes the program more user-friendly. For example, look at the following program.
Dim name As String
Dim age As Byte
Console.Write("What is your name? ")
name = Console.ReadLine()
Console.Write("What is your age? ")
age = Console.ReadLine()
Console.Write("Wow, you are already" & age & "years old," & name & "!")
Python
In Python, data input is accomplished using one of the following statements:
#Read a string from the keyboard
var_name_str = input([prompt])
#Read an integer from the keyboard
var_name_int = int(input([prompt]))
#Read a real from the keyboard
var_name_float = float(input([prompt]))
where
prompt
is the prompt message to be displayed. It can be either a variable or a string enclosed in single or double quotes. The argumentprompt
is optional.var_name_str
can be any variable of type string.var_name_int
can be any variable of type integer.var_name_float
can be any variable of type float.
Notice: Functions int()
and float()
are discussed later.
The following code fragment lets the user enter his or her name and age.
name = input()
age = int(input())
print("Wow, you are already", age, "years old,", name, "!")
However, it could be even better if, before each data input, a “prompt” message is displayed. This makes the program more user-friendly. For example, look at the following program.
name = input("What is your name? ")
age = int(input("What is your age? "))
print("Wow, you are already", age, "years old,", name, "!")
Now when a user executes your program he or she knows exactly what to enter!
The example that follows is equivalent to the previous one.
print("What is your name? ", end = "")
name = input()
print("What is your age? ", end = "")
age = int(input())
print("Wow, you are already", age, "years old,", name, "!")
Notice: In Python, it is recommended to display prompt messages using the
input()
statement.
The corresponding flowchart fragment looks like this.
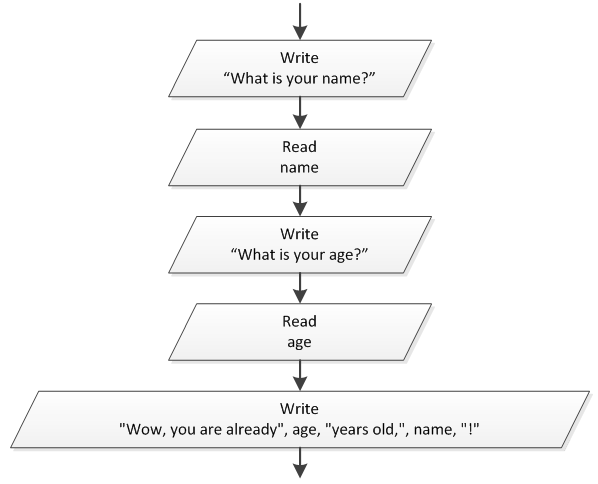