The first thing you must do is create a new C# project. You have already added a folder to the workspace (see here). It is probably named “VS Code CS Projects”. Click on the “Explorer” button in the Activity Bar on the left and select the folder “VS Code CS Projects”.
Hit the CTRL + SHIFT + P key combination to open the Command Palette, and run the “.NET: New Project…” command, as shown in Figure 1.
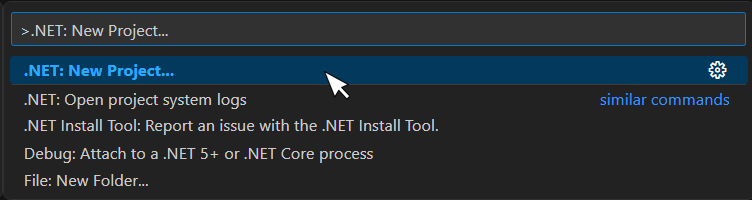
From the drop down list that opens, select “Console App”.
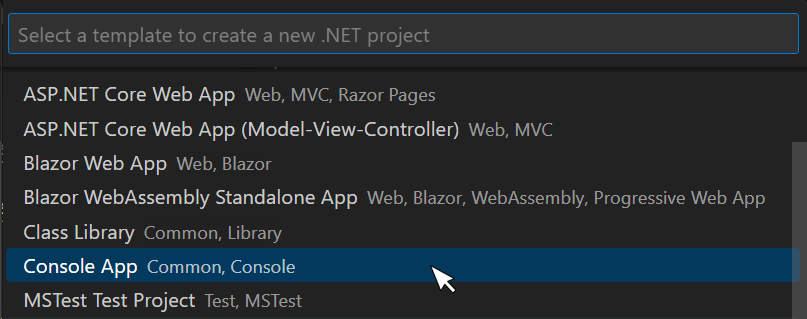
In this step, Visual Studio Code asks you for the name of the new project. Type “testingProject” as shown in Figure 3.

And finally, choose the folder “VS Code CS Projects” where your project will be created.

Select the “Program.cs” file. Your Visual Studio Code environment should look like the one in Figure 5.
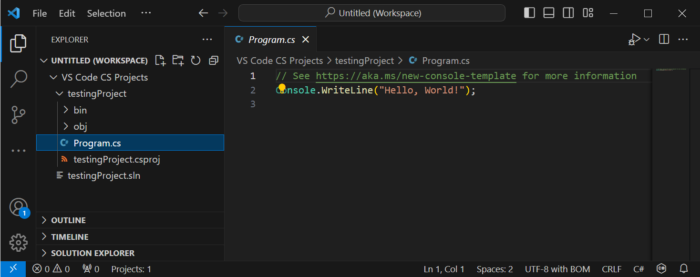
You have just seen how to create a new C# console project. Let’s now write the following (terrifying, and quite horrifying!) C# program and try to execute it.
Console.WriteLine("Hello, World!"); Console.WriteLine("Hallo, Welt!"); Console.WriteLine("Bonjour, le Monde!");
As you may have noticed, the first line that already exists in Visual Studio Code is a comment, so you can just delete that line. In the second line, the statement that displays the English message “Hello, World!” has been already entered for you automatically. Place your text cursor at the end of that line and hit the “Enter ⤶” key. Let’s try to type the second statement, the one that displays the German message “Hallo, Welt!”. Type only the first character, “C”, from the Console
keyword by hitting the “C” key on your keyboard. A popup window appears, as shown in Figure 6. This window contains all available C# statements, and other items that begin with the character “C”.
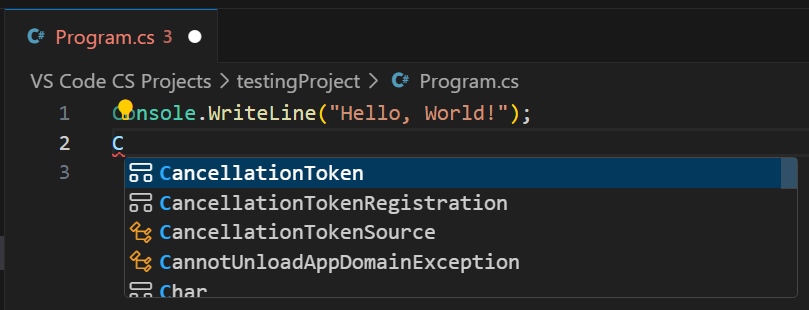
Notice: You can highlight a selection by using the up and down arrow keys on your keyboard.
Type the second character, “o”, from the Console
keyword. Now the options have become fewer. Select the option “Console” using the down arrow key from your keyboard (if necessary), as shown in Figure 7.
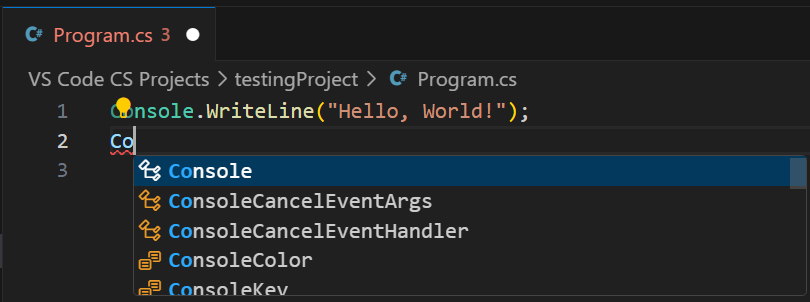
Hit the period “ . ” key. The keyword Console
is automatically entered into your program. Select the “WriteLine” option as shown in Figure 8 and hit the “Enter ⤶” key.
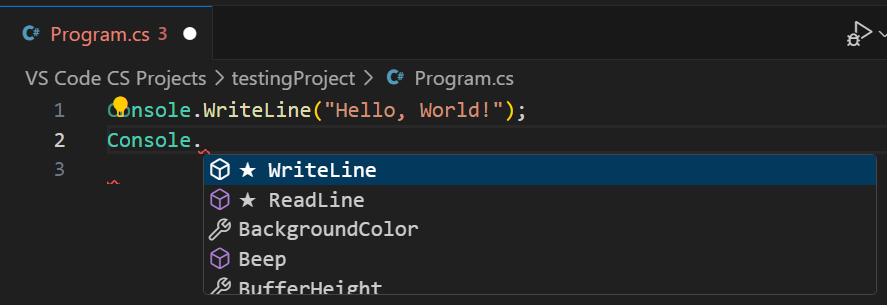
Complete the statement by writing Console.WriteLine("Hallo, Welt!");
Then, continue typing the rest of the C# program (as shown in Figure 9). Save the changes that you have just made. Your Visual Studio Code environment should look like this.
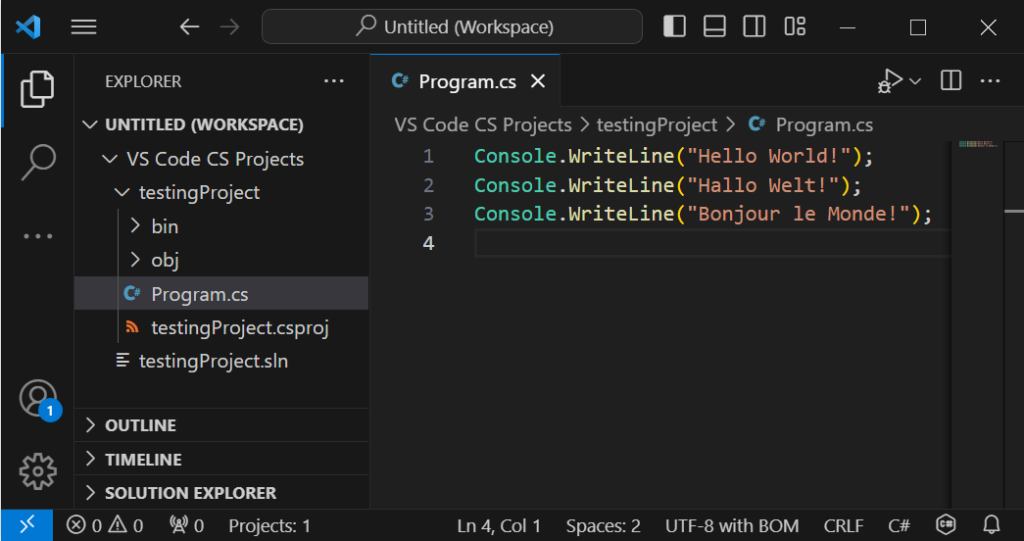
And now, let’s try to execute it! From the main menu, select “Run → Run Without Debugging”.
The C# program executes, and the output is displayed in the “Terminal” window, as shown in Figure 10.
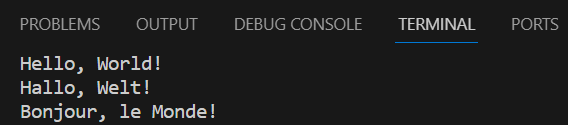
Notice: Alternatively, you can execute a file by hitting the CTRL + F5 key combination.
Congratulations! You have just written and executed your first C# program!
Notice: You should always save your changes before executing a program. To do so, from Visual Studio’s main menu you can select “File → Save” or hit the “CTRL + S” key combination!
Now let’s write another C# program, one that prompts the user to enter their name. Type the following C# program into Visual Studio Code and hit CTRL + F5 to execute the file.
string name; Console.Write("Enter your name: "); name = Console.ReadLine(); Console.WriteLine("Hello " + name);
Once you execute the program, the message “Enter your name:” is displayed in the “Terminal” window. The program waits for you to enter your name, as shown in Figure 11.
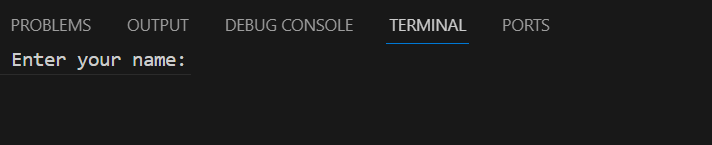
Type your name and hit the “Enter ⤶” key. Once you do that, your computer continues to execute the rest of the statements. When execution is complete, the final output is as shown in Figure 12.
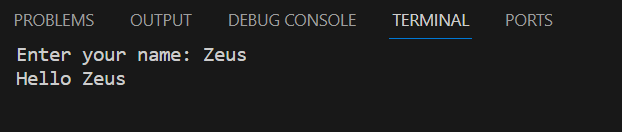