The first thing you must do is create a new PHP file. You have already added a folder to the workspace (see here). It is probably named “VS Code PHP Projects”. Click on the “Explorer” button in the Activity Bar on the left and select the folder “VS Code PHP Projects”. In the Side Bar that opens, click on the “New File”
option and create a file named “testingFile.php” (as shown in Figure 1).
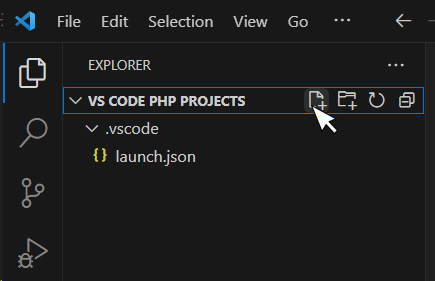
Now, write the following (terrifying, and quite horrifying!) PHP script.
<?php echo "Hello World\n"; echo "Hallo, Welt!\n"; echo "Bonjour, le Monde!\n"; ?>
Type <?
and in the popup window that opens, hit the “Enter ⤶” key. The opening tag <?php
is automatically entered into your script.
Now, let’s try to type the echo
statement, the one that displays the English message “Hello, World!”. Type only the first character, “e”, from the echo
keyword by hitting the “e” key on your keyboard. A popup window appears, as shown in Figure 2. This window contains all available PHP statements, and other items that begin with the character “e”.
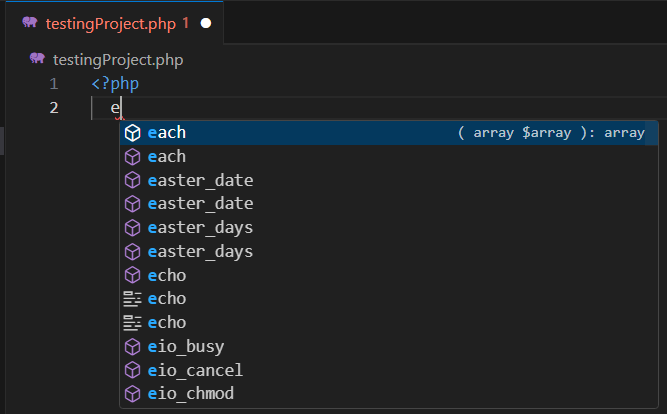
Notice: You can highlight a selection by using the up and down arrow keys on your keyboard.
Type the second character, “c”, from the echo
keyword. Now the options have become fewer. Select the option “echo” using the down arrow key from your keyboard (if necessary), as shown in Figure 3.
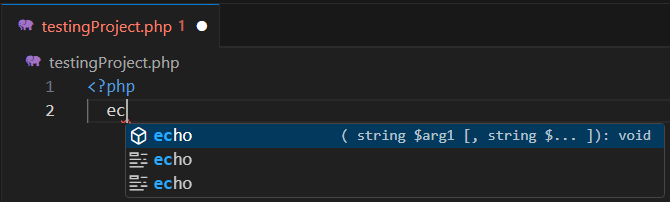
Hit the “Enter ⤶” key. The keyword echo
is automatically entered into your script. Complete the statement by writing echo "Hello, World!");
Then, continue typing the rest of the PHP script (as shown in Figure 4). Save the changes that you have just made. Your Visual Studio Code environment should look like this.
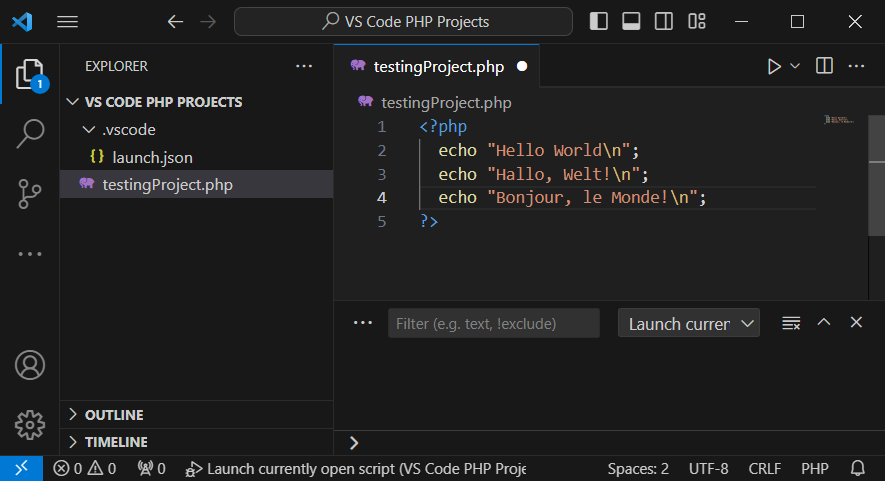
And now, let’s try to execute it! From the main menu, select “Run → Start Debugging”. The PHP script executes, and the output is displayed in the “Console” window, as shown in Figure 5.
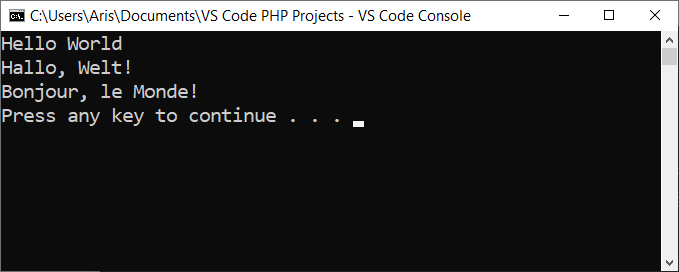
Notice: Alternatively, you can execute a file by hitting the F5 key.
Congratulations! You have just written and executed your first PHP script!
Now let’s write another PHP script, one that prompts the user to enter their name. Type the following PHP script into Visual Studio Code and hit F5 to execute the file.
<?php $name = readline("Enter your name: "); echo "Hello", $name, "\n"; echo "Have a nice day!\n"; ?>
Once you execute the script, the message “Enter your name:” is displayed in the “Console” window. The script waits for you to enter your name, as shown in Figure 6.
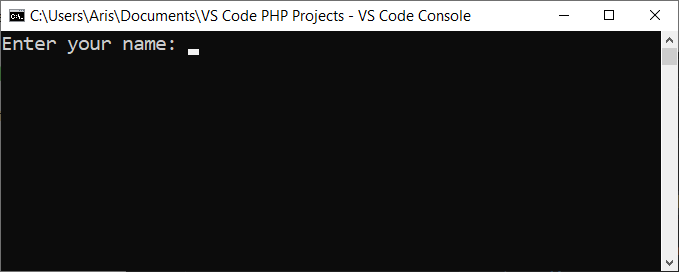
Type your name and hit the “Enter ⤶” key. Once you do that, your computer continues to execute the rest of the statements. When execution is complete, the final output is as shown in Figure 7.
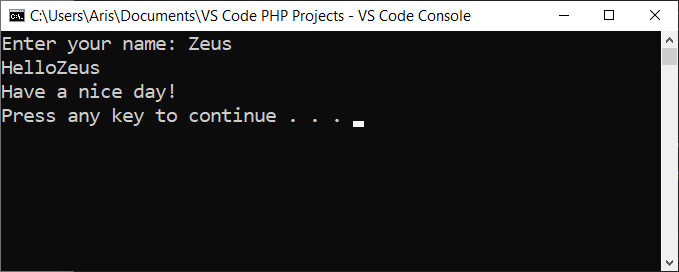